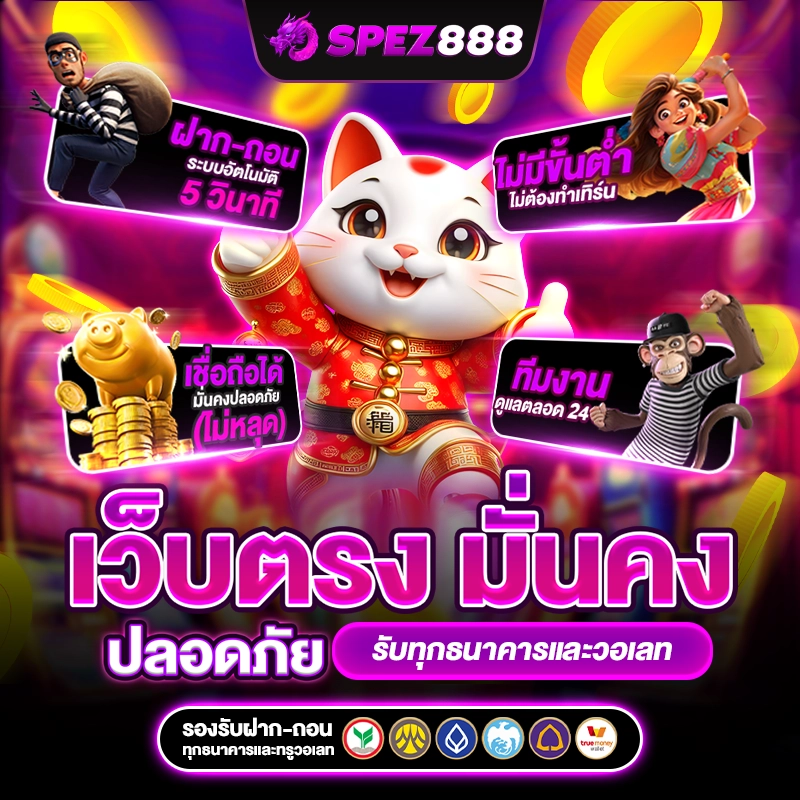
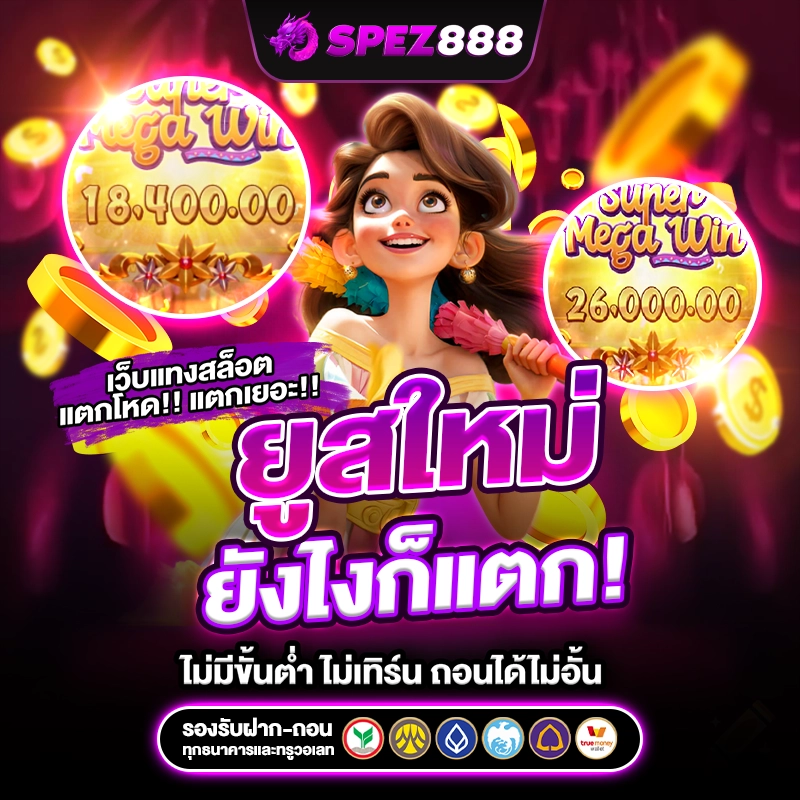
สล็อตเว็บตรง เว็บตรงที่ให้การอัปเดตเกมแตกง่าย แตกหนัก เกมใหม่ ๆ อย่างต่อเนื่อง เพื่อมอบความบันเทิงไม่รู้จบให้กับนักพนันตลอดทั้งวัน เมื่อเข้าถึงสล็อตเว็บตรง แตกง่าย ไม่มีขั้นต่ำ ที่มีคุณภาพอย่างเว็บนี้ คุณสามารถมั่นใจได้ถึงมาตรฐานสากล ที่จะช่วยให้การลงทุนในทุกเกมบนเว็บมั่นใจมากยิ่งขึ้น พร้อมให้อัตราการเดิมพันเกมสล็อต เว็บตรง แตกหนัก ที่เป็นประโยชน์ต่อผู้เล่นเกมอย่างมาก ด้วยเกมตรง ลิขสิทธิ์แท้ เว็บตรง 100% จากทางค่ายเกม มีช่องทางการเข้าถึงบริการบนเว็บที่ได้รับการออกแบบมาให้ใช้งานง่าย ไม่ซับซ้อน เข้าเล่นเกมสล็อตบนเว็บตรงแห่งนี้ได้ง่าย มีแจ็คพอตแตกดีจริง และมอบเล่นเกมเว็บสล็อตใหม่ล่าสุด ที่ดีที่สุดให้กับสมาชิกทุกคน สามารถเข้าถึงการอัปเดตล่าสุดในปี 2024 ได้โดยไม่มีขั้นต่ำ รับรองระบบการเล่นเกมสล็อตเว็บตรง อันดับ 1 ที่ราบรื่นและสนุกสนานอย่างมาก
สล็อตเว็บตรง เว็บตรงทำไมถึงได้รับความนิยมอย่างมากในไทย เพราะเป็น SLOT เว็บตรง ของแท้ 100% ได้การรับรองจากองค์กรสากลมาแล้วเป็นที่เรียบร้อย เว็บพนันรุ่นใหม่มาแรง สล็อตเว็บตรง แตกง่าย บริการต่อเนื่องและดีจริง 24 ชั่วโมง ไม่มีปิดหนี ให้ระบบสล็อตเว็บตรง ฝาก-ถอน true wallet ไม่มีธนาคาร ไม่มีขั้นต่ำ พร้อมมอบฟังก์ชันการเดิมพันที่สะดวกและหลากหลายที่สุดให้กับนักพนัน
เว็บสล็อตวอเลท หลายธีมจากหลายค่ายชั้นนำ ให้นักพนันลงทุนได้อย่างง่ายดาย จึงเป็นตัวเลือกการลงทุนบนเกมเว็บตรงไม่ผ่านเอเย่นต์ ที่หลากหลายจากผู้ให้บริการที่มีชื่อเสียงระดับโลก เว็บตรงที่ดีที่สุด การันตีมาตรฐานสากล ให้ระบบ API ที่มีคุณภาพมากที่สุด ผู้เล่นเกมจึงสามารถเข้าร่วมและใช้บริการได้อย่างมั่นใจ นอกจากนี้ ยังมีเกมเกมสล็อต ให้เลือกเล่นมากมาย เมื่อสมัครเข้ามาแล้วเล่นเกมได้ทั้งวันอย่างไม่มีเบื่อแน่นอน
สล็อตเว็บตรง รับประกันการเข้าถึงเกมที่รวดเร็ว ปลอดภัย ด้วยระบบอัตโนมัติที่เชื่อถือได้ พร้อมการอัปเดตระบบอัตโนมัติให้มีประสิทธิภาพสูงเสมอ ทำให้ผู้เล่นเว็บสล็อต สามารถวางเดิมพันและทำธุรกรรมได้อย่างง่ายดาย มีความคุ้มค่า รวดเร็วทันใจ ทำได้ด้วยตัวเอง สามารถฝากและถอนเงินได้ภายในเวลาเพียง 5 วินาที โดยไม่มีข้อกำหนดขั้นต่ำ ไม่ต้องรอแอดมินของทางเว็บให้เสียเวลา
ส่วนของเกมสล็อตเว็บตรง แตกง่าย จะมีการอัปเกรดฟีเจอร์ใหม่มากมาย มาให้ผู้เล่นได้เพลิดเพลินและลงทุนอย่างมีกำไร จึงเป็นสล็อตเว็บตรง ไม่ผ่านเอเย่นต์อันดับ 1 ด้านงานบริการ พร้อมช่วยให้สร้างรายได้ในอัตราที่สูงขึ้น ทำให้ผู้เล่นมีโอกาสทำกำไรมากมาย มีโอกาสชนะสูงกว่าเดิม จึงเป็นแพลตฟอร์มเว็บตรงแตกบ่อยที่มีความหลากหลายที่สุดสำหรับผู้ที่ชอบเล่นเกมสล็อต
หากคุณกำลังมองหาข้อดีของการวางเดิมพันในเกมสล็อตวอเลท บน สล็อตเว็บตรง แนะนำ 3 ข้อดีต่อไปนี้ที่จะทำให้คุณหลงรักเว็บ SLOT เว็บตรงที่ดีที่สุด สามารถสร้างความมั่นใจให้กับนักพนัน ในการเลือกลงทุนเกมสล็อต RTP เกิน 90% ที่ชอบเล่นได้ง่ายขึ้นด้วยเงินทุนต่ำ โดยระบบสล็อตวอเลทเป็นการโอนฝากถอนเงินผ่านแอปพลิเคชั่น True Money Wallet ที่ให้ความรวดเร็วทันใจมาก ปลอดภัยสูง ซึ่งข้อดีของการใช้บริการสล็อตทรูวอเลทเพิ่มเติม มีดังนี้
การฝากและถอนเงินผ่าน True Wallet ได้รับการดำเนินการอย่างมีประสิทธิภาพและปลอดภัย รับประกันบริการด้วยระบบอัตโนมัติที่ช่วยให้ทำธุรกรรมได้อย่างรวดเร็วและเข้าถึงได้ตลอดเวลา ไม่มีขั้นต่ำ งบแค่ไหนก็โอนมาเล่นเกมได้สบาย ไม่ต้องใช้บัญชีธนาคาร ทำให้ผู้ใช้งานสล็อตเว็บตรงแตกหนัก ระบบวอเลททุกคนได้รับที่ราบรื่นมาก
เมื่อชนะเกมSLOT เว็บตรง แล้วรับเงินรางวัล พร้อมทำยอดเทิร์นโปรครบ! รับรางวัลเต็มจำนวนทันทีเมื่อคุณใช้บริการ สล็อตเว็บตรง สามารถรับโปรโมชั่นสล็อตวอเลทได้บ่อยครั้ง แจกให้กับสมาชิกใหม่และสมาชิกเก่าอยู่ตลอด ทำให้คุณสามารถเลือกและรับฟรีได้ทุกวัน โดยที่เงื่อนไขของโปรโมชั่นไม่มีความยุ่งยากเลยแม้แต่น้อย
สำหรับผู้ที่เล่นเกมพนันเป็นประจำและใช้งาน Slot wallet เมื่อใช้บริการเว็บสล็อตอันดับต้น ๆ ของไทยอย่าง สล็อตเว็บตรง รับเงินคืนรายวันได้เลย รวมอยู่ในการคืนยอดเสียให้กับผู้เล่นด้วยเปอร์เซ็นต์ที่คุ้มค่ามาก คุณจะได้รับความพึงพอใจและความสนุกสนานไปพร้อมกัน
สล็อตเว็บตรง คือ เว็บตรงสล็อตที่ไม่ต้องผ่านเอเย่นต์ ทำให้เป็นตัวเลือกการลงทุนยอดนิยมในปี 2024 ด้วยรูปแบบการเดิมพันที่เรียบง่ายและตรงไปตรงมา ทดลองเล่นสล็อต ด้วยระบบทดลองใช้ฟรี เพื่อให้คุณศึกษากฎและรูปแบบการเล่นอย่างละเอียด เว็บสล็อตวอเลท เว็บตรง จึงได้รับความนิยมอย่างสูง ทั้งในหมู่ผู้เล่นใหม่และมืออาชีพ ให้บริการตลอด 24 ชั่วโมง รับประกันเป็นเว็บแท้ เว็บตรง เว็บสล็อตใหม่ล่าสุด คุณภาพและเชื่อถือได้ ซึ่งจ่ายเงินรางวัลอย่างรวดเร็วภายในไม่กี่นาที
สล็อตเว็บตรงไม่ผ่านเอเย่นต์ แพลตฟอร์มมีความปลอดภัย ใช้งานง่าย และรองรับธุรกรรมต่าง ๆ เพื่อตอบสนองความต้องการของนักพนันยุคใหม่ พร้อมเสิร์ฟ! ความสนุกแบบไร้ขีดจำกัด เล่นเกมสล็อตแตกง่ายอย่างสะดวกสบาย ทางเว็บปั่นสล็อต ทุ่มเทเพื่อส่งมอบความสุขให้กับคุณบนหน้าจอโทรศัพท์มือถือ ไม่ว่าคุณจะใช้ระบบปฏิบัติการใด ไม่ว่าคุณจะอยู่ที่ไหนและเมื่อใดก็ตาม คุณสามารถทำกำไรจาก SLOT AUTO ได้อย่างง่ายดายตลอดทั้งวัน
เล่นเกมสล็อตบนเว็บดัง เว็บที่น่าสนใจ จากการแนะนำของ เว็บตรงสล็อต ทำให้นักพนันสามารถทำกำไรจากเกมทุกประเภทได้ง่ายขึ้น มีระบบสล็อตเว็บตรง ฝาก-ถอน true wallet ไม่มีธนาคาร ไม่มีขั้นต่ำ จึงไม่ต้องมีบัญชีธนาคาร สร้างรายได้ในอัตราสูงได้ บริการเกมสล็อตที่รับประกันการลงทุนที่สม่ำเสมอ มอบเกมเว็บสล็อต ใหม่ล่าสุด 2024 ที่ดีที่สุดให้กับผู้เล่น รวมถึงโอกาสในการคว้ารางวัลแจ็คพอตได้อย่างง่ายดาย โดยมี 6 เว็บสล็อตสุดปังที่ทำให้การสมัครสมาชิกคุ้มค่าแน่นอน คือ
เกมสล็อตบนเว็บ Spez777 เป็นเกมที่เล่นง่าย แตกหนัก แตกดีจริง เว็บตรง100% ได้รับความนิยมอย่างมากในปัจจุบัน เนื่องมาจากรูปแบบการเดิมพันสล็อตฝากถอน true wallet เว็บตรง ที่เข้าใจง่าย เป็นที่ชื่นชอบของนักพนันทั้งชาวไทยและต่างชาติ สมาชิกสามารถวางเดิมพันเกมสล็อตบนเว็บได้อย่างอิสระ สนุกสนานไปกับการเล่นเกมที่ดีที่สุด พร้อมเปิดให้ใช้งานได้ตลอด 24 ชั่วโมง ไม่มีวันหยุด ไม่ว่าคุณจะวางเดิมพันเมื่อใด คุณก็จะมีช่วงเวลาที่ยอดเยี่ยมบนเว็บนี้อย่างแน่นอ
Spez168 เว็บพนันรุ่นใหม่ เว็บตรงมาแรง ให้บริการด้วยระบบปั่นสล็อตที่ยอดเยี่ยม มีความทันสมัยทั้งเว็บ ใช้งานระบบอัตโนมัติที่มีความรวดเร็ว คัดเกมที่เล่นแล้วแตกรางวัลดีจริงมาให้เล่นไปตลอดทั้งวัน ระบบฝากถอนและการทำธุรกรรมมีความเร็ว มีความหลากหลาย เลือกได้ตามความถนัด พร้อมทำให้การเล่นเกมเว็บสล็อตตรงทุกวันของคุณ มีความสะดวกสบายอย่างสูงสุด ชนะเดิมพันแล้วรับเงินเลย การใช้งานต่าง ๆ บนเว็บง่ายดาย เมื่อสมัครมาแล้วจึงไม่ต้องกังวล
สัมผัสเกมสล็อตชั้นนำจากทั่วโลกบนเว็บตรงไม่ผ่านเอเย่นต์ 1 บาท ก็ถอนได้ Sepz666 ทุกเกมได้รับความนิยมอย่างสูง โดยมีตัวเลือกให้มากกว่า 500 รายการ เมื่อสมัครสมาชิกวันนี้! พร้อมรับเครดิตฟรีได้เลย เลือกจากเกมสล็อตคุณภาพเยี่ยมที่คัดสรรมาจากค่ายเกมที่ดีที่สุดในเอเชีย ออกแบบมาเพื่อมอบความบันเทิงให้กับผู้เล่น เข้าร่วมกับ เว็บสล็อต ทั้งหมด ผู้ให้บริการเกมสล็อตชั้นนำและเพลิดเพลินไปกับโบนัสกับแจ็คพอตมากมาย หากคุณสนใจที่จะลองเล่น สามารถเข้าถึงได้จากเว็บตรงแห่งนี้อย่างง่ายดาย หมุนเกมสล็อตและเริ่มรับเงินวันนี้ได้เลย
Punslot444 นำเสนอเกมสล็อตผ่านทุกอุปกรณ์ ให้ลูกค้าได้สัมผัสกับความตื่นเต้นของการเดิมพันสล็อตด้วยเกมคุณภาพเยี่ยม จากผู้ให้บริการเกมชั้นนำทั่วโลก เกมเล่นง่าย เว็บสล็อต อันดับ 1 จ่ายเงินรางวัลใหญ่รวดเร็ว สนุกไปกับมิติใหม่ของการเล่นเกมสล็อตด้วยเงินรางวัลจริง ทำให้คุณเล่นสล็อตเว็บตรง ไม่ผ่านเอเย่นต์ไม่มีขั้นได้อย่างสะดวกสบายจากบ้านของคุณเองหรือที่ใดก็ได้ ทุกเมื่อที่ต้องการ พร้อมบริการเกมสล็อตบนมือถือที่จะทำให้คุณลืมเกมสล็อตแบบเดิม ๆ ไปได้เลย สามารถเข้าถึงเกมสล็อตได้ตลอด 24 ชั่วโมง โดยมีระบบอัตโนมัติ เพื่อเพิ่มความสะดวกสบายให้กับผู้เล่นเกมอย่างเต็มที่
เว็บสล็อต Punslot789 ได้มาตรฐานสากลภายในเว็บ พัฒนาระบบบริการสล็อตเว็บตรงทรูวอเลท ได้อย่างยอดเยี่ยมให้กับลูกค้าทุกคนอย่างต่อเนื่อง ซึ่งช่วยให้การเล่นเกมสล็อตของคุณเสถียรและมีประสิทธิภาพ นอกจากนี้ ระบบยังมีคุณภาพสูงด้วยกราฟิกแบบ Full HD และเอฟเฟกต์เสียงต่าง ๆ ที่จะช่วยเพิ่มความตื่นเต้นในการเล่นเกม คุณสามารถเพลิดเพลินกับความสนุกแบบไร้ขีดจำกัดและไม่สะดุด ด้วยเกมมากมายกว่า 1,000 เกม จากผู้ให้บริการเกมพนันชั้นนำทั่วโลก ผู้ให้บริการมีชื่อเสียงในอุตสาหกรรมเกมพนันออนไลน์และได้รวบรวมไว้ในเว็บตรง 100% เว็บเดียว เพื่อความสะดวกของสมาชิกทุกคน
เกมสล็อตเว็บตรง 100% ไม่เพียงแต่เป็นความท้าทายที่น่าตื่นเต้นเท่านั้น แต่ยังเป็นโอกาสในการยกระดับตัวเอง ขึ้นสู่สถานะของผู้ชนะที่มีเงินกำไรจำนวนมาก เปลี่ยนแปลงชีวิตของคุณได้ทันที หากคุณพร้อมที่จะเผชิญกับความท้าทายในวงการพนันที่น่าตื่นเต้น อย่าพลาดการเข้าร่วมเล่นเกมกับเว็บสล็อต DRAGON444 ซึ่งเป็นตัวเลือกยอดนิยม ไม่เพียงแต่เป็นผู้ให้บริการเกมพนันชั้นนำเท่านั้น แต่ยังมีชื่อเสียงในเรื่องการเดิมพันแล้วได้เงินจริง ได้กำไรตามต้องการแน่นอน
สล็อตเว็บตรง เสนอ 3 เทคนิคที่พิสูจน์แล้วสำหรับการวางเดิมพันในเกมสล็อต ซึ่งรับประกันความแม่นยำสูงสุดในการสร้างรายได้ให้กับนักพนัน แพลตฟอร์มนี้จะช่วยให้เข้าถึงเกมสล็อตได้ง่ายขึ้น และมีตัวเลือกมากมายสำหรับผู้ใช้ มั่นใจได้ว่านักลงทุนจะได้รับประโยชน์จากรูปแบบเกมที่แตกต่างกัน เล่นสนุกไม่มีเบื่อ เว็บสล็อตแท้ใช้งานง่ายและสร้างกำไรให้กับนักพนันทุกคน พร้อมให้ 3 เทคนิคชนะเกมสล็อต ช่วยเพิ่มโอกาสชนะ เสริมความมั่นใจให้กับผู้เล่นเกมมากขึ้น คือ
เทคนิคโปรแกรม AI เล่นเกมสล็อตจาก เว็บตรง มีความหลากหลายและมีรูปแบบการใช้งานที่แตกต่างกัน เพียงเลือกเทคนิคที่เหมาะสมที่สุดกับตัวคุณเอง และใช้ร่วมกับการเล่นเกมSLOT เว็บตรง จะเพิ่มประสิทธิภาพในการสร้างผลกำไรได้หลายเท่าเลยทีเดียว
เกมสล็อตบางเกมอาจไม่มีโบนัส จึงควรเข้าถึงข้อมูลโดยละเอียดเกี่ยวกับแต่ละเกมผ่านรีวิวเกมบนเว็บหลัก หรือหน้าการตั้งค่าของเกม เมื่อเข้าสู่เว็บสล็อต คุณจะสามารถตัดสินใจได้ว่าเกมใดมีคุณสมบัติโบนัสพิเศษหรือฟีเจอร์คุ้มค่า วิธีนี้จะช่วยให้คุณเลือกเกมสล็อตที่เหมาะสมที่จะเล่นเว็บสล็อตตรงไม่ผ่านเอเย่นต์ และเพิ่มโอกาสในการชนะรางวัลใหญ่ มั่นใจได้เลยว่าคุณจะมีโอกาสเพลิดเพลินและรับผลกำไรจากการเล่นเกมอย่างเต็มที่
ช่วงเวลาการเล่นเกมสล็อต หรือช่วงโบนัสไทม์ เป็นอีกปัจจัยสำคัญที่สามารถเพิ่มโอกาสในการทำกำไรได้อย่างมาก สล็อตเว็บตรง จึงแนะนำให้เล่นสล็อตในช่วงเวลาโบนัสจากตารางเวลาที่ถูกจัดมาอย่างดี โดยระบุช่วงเวลาที่เกมสล็อตมีอัตราการจ่ายแจ็คพอตสูงสุด หากเล่นในช่วงเวลาที่แนะนำ คุณสามารถเพิ่มโอกาสในการทำกำไรสล็อตเว็บตรง ฝาก-ถอน true wallet ไม่มีขั้นต่ำได้มากถึงหลักล้านบาทเลยทีเดียว
ค้นพบข้อดีของ สล็อตเว็บตรง ไม่ต้องผ่านเอเย่นต์ สำหรับผู้ที่กำลังพิจารณาหรือต้องการรายละเอียดเพิ่มเติม ได้รวบรวมไฮไลท์ของแหล่งเดิมพันสล็อตที่เล่นง่าย เพื่อเป็นแนวทางในการตัดสินใจ ข้อมูลแต่ละส่วนมีจุดแข็งและข้อดีเฉพาะตัวที่ตอบโจทย์ลูกค้าได้อย่างเต็มที่ รับรองว่าไม่มีเว็บใดที่จะดูแลลูกค้าได้ดีเท่านี้ ไม่เพียงแต่ให้บริการตลอด 24 ชั่วโมงเท่านั้น แต่ยังมีฟีเจอร์ที่เหมาะสมที่สุดสำหรับการลงทุนอีกด้วย โดยสล็อต เว็บตรงแตกหนัก มี 3 ข้อดีที่น่าสนใจ ดังนี้
ทดลองเล่นสล็อต โหมดที่มีให้บริการบนสล็อตแท้ เปิดให้เล่นเกมได้ตลอด 24 ชั่วโมง ถือเป็นฟีเจอร์ที่มีประโยชน์อย่างยิ่ง สำหรับนักลงทุนหน้าใหม่และนักพนันที่มีประสบการณ์ สามารถใช้บริการเกมทดลองเพื่อฝึกฝนฝีมือ โดยไม่ต้องเสี่ยงเงินทุนของตนเอง นอกจากนี้ นักพนันยังสามารถใช้บริการนี้ เพื่อฝึกฝนทักษะและยกระดับการเล่นเกมสล็อต ไปสู่ระดับมืออาชีพได้ง่ายขึ้น แต่ละเกมสล็อตเว็บตรง แตกง่าย มาพร้อมกับกราฟิกที่สวยงาม และเอฟเฟกต์ที่น่าประทับใจ
สล็อต เว็บตรง รองรับทุกอุปกรณ์บนทุกระบบปฏิบัติการ ไม่ว่าคุณจะเล่นผ่านระบบ iOS หรือ Android ก็พร้อมที่จะมอบความสนุกสนานให้กับคุณบนหน้าจอโทรศัพท์มือถือของคุณ สำหรับผู้ที่ไม่สะดวกจะเล่นบนหน้าจอโทรศัพท์มือถือ คุณสามารถเข้าสู่ระบบและเล่นสล็อต เว็บตรงแตกหนัก บนคอมพิวเตอร์ได้เลย สลับระหว่างอุปกรณ์ต่าง ๆ ได้อย่างสะดวก โดยไม่ต้องยุ่งยากกับการเปลี่ยนบัญชีผู้ใช้งานให้เสียเวลา แพลตฟอร์มคอมพิวเตอร์รองรับ Windows เวอร์ชันต่าง ๆ ทำให้คุณมีโอกาสมาเดิมพันเพื่อความสนุกที่ไร้ขีดจำกัด
สล็อตเว็บตรง เสนอการเดิมพันเริ่มต้นเพียง 1 บาท เหมาะกับผู้ที่มีงบประมาณจำกัด แม้ว่าคุณจะลงทุนเพียงเล็กน้อย 10 บาท หรือ 100 บาท คุณก็มีโอกาสที่จะเล่นและมีโอกาสชนะรางวัลแจ็คพอตภายในวันเดียว แต่ละเกมปั่นสล็อต เว็บตรงมีอัตราการชนะอย่างได้มาตรฐาน ทำให้คุณมีโอกาสลองเสี่ยงโชคกับการหมุนเกมทุกครั้งจนถึงวินาทีสุดท้าย สล็อต เว็บตรง ขั้นต่ำ 1 บาท ถือเป็นเว็บสล็อตที่ดีที่สุด มอบวิธีที่ง่ายที่สุดในการทำเงินให้กับคุณ
นำเสนอ 6 ค่ายเกมยอดนิยมจากการแนะนำของเว็บแท้ที่มีอัตราการชนะสูงสุด ค่ายเกมเหล่านี้แสดงผลลัพธ์อย่างรวดเร็ว ทำให้เป็นเกมที่ฮิตที่สุดบนสล็อต เว็บตรง ให้โอกาสในการทำเงินที่ดีที่สุดในปี 2024 แต่ละเกมมีรูปแบบการเล่นที่เรียบง่าย ทำให้ผู้เล่นใหม่ลงทุนได้อย่างมั่นใจ สำหรับนักพนันที่กำลังมองหาเกมสล็อตที่ให้ทั้งทำกำไรและสนุกสนาน ขอแนะนำให้ลองเล่นเกมจาก 6 ค่ายเกมต่อไปนี้
เกม PG SLOT ไม่เพียงแต่ทำกำไรได้สูงเท่านั้น แต่ยังมอบการเล่นเกมที่ราบรื่นและสนุกสนานอีกด้วย มีระบบปฏิบัติการที่เสถียรสูง ช่วยให้ผู้เล่นทุกคนเล่นเกมได้อย่างราบรื่น ไม่มีข้อผิดพลาดหรือการหยุดชะงัก การเดิมพันทุกครั้งจึงรับประกันได้ว่าสนุกสนาน มั่นใจได้เลยว่าทุกคนจะได้สัมผัสกับการเล่นเกมสล็อต เครดิตฟรี 50 ไม่ต้องฝากก่อน ไม่ต้องแชร์ ยืนยันเบอร์โทรศัพท์ ที่สดใหม่และน่าตื่นเต้นบนเว็บตรง ซึ่งสล็อตนั้นชนะได้ง่ายและไม่มีขั้นต่ำ
ผู้ให้บริการ Jili Slot ค่ายน้องใหม่มาแรง นำเสนอเกมสล็อตหลากหลายประเภทบน สล็อตเว็บตรง แพลตฟอร์มมีเกมล่าสุดที่นำเข้าโดยตรงจากต่างประเทศ ใช้ประโยชน์จากเทคโนโลยีอินเทอร์เน็ต 5G เพื่อมอบความราบรื่นให้กับผู้เล่นเกม ด้วยกราฟิกที่รองรับความละเอียด 4K ผู้เล่นสามารถเพลิดเพลินกับการเล่นเกมที่ไม่สะดุด ซึ่งทั้งสวยงามและน่าดึงดูด แนวทางที่สร้างสรรค์ว่าจะให้ผู้เล่นได้รับความบันเทิง ด้วยรูปแบบการเล่นเกมสล็อต เว็บตรงไม่ผ่านเอเย่นต์ไม่มีขั้นต่ำที่น่าตื่นเต้น ภาพสดใส และเอฟเฟกต์เสียงที่สมจริง
918Kiss คือ เกมที่ดีที่สุดที่คุณสามารถลองเล่นได้ โดยอดีตมีชื่อว่า SCR888 การเลือกเกมไปเล่นมีความเป็นอิสระ และระบบการลงทะเบียนสมัครง่ายดาย เกมดีลเลอร์สดได้รับการปรับปรุงและปรับให้เหมาะสมต่อการเล่นเกม สล็อต ทดลองเล่นฟรี ถอนได้ มีความหลากหลายสูง ให้บรรยากาศเหมือนในคาสิโนจริง เดิมพันร่วมกันกับ สล็อต เว็บตรง แล้วชนะไปได้อย่างมั่นใจ
สัมผัสการเล่นเกมสล็อตขั้นสุดยอดกับ Slotxo ซึ่งมีเกมให้เลือกเล่นกว่า 200 เกม บน สล็อตเว็บตรง ให้สมาชิกได้เพลิดเพลิน มุ่งมั่นที่จะมอบบริการชั้นยอดและเกมสล็อตที่ดีที่สุด รวมถึงเกมยอดนิยมที่รับรองว่าจะทำให้คุณเพลิดเพลินและมีโอกาสคว้าโบนัสแจ็คพอตได้ง่ายขึ้น เกมสล็อต ฝาก-ถอน true wallet ไม่มี บัญชีธนาคารมีคุณภาพสูง สามารถเล่นได้ทั้งบนคอมพิวเตอร์และอุปกรณ์พกพา เกมมีโบนัสแจกบ่อยครั้ง แตกแจ็คพอตมหาศาล และให้การจ่ายเงินบ่อยครั้ง ทำให้คุ้มค่าและสนุกสนานจริง
Pragmatic Play เป็นผู้ให้บริการเกมสล็อตชื่อดังบน สล็อตเว็บตรง หรือรู้จักกันในชื่อ PP Slot เป็นหนึ่งในผู้ให้บริการเกมที่ให้ผลตอบแทนสูงสุด โดยเสนอผลตอบแทนที่สูงกว่าเมื่อเทียบกับผู้ให้บริการรายอื่น ด้วยอัตราการจ่ายเงินสูง ให้โบนัสที่คุ้มค่า ชนะได้ง่าย และมีเกมเว็บสล็อตตรงให้เลือกเล่นมากกว่า 100 เกม โดดเด่นด้วยรูปแบบเกมสล็อตแนวนอนที่ไม่เหมือนใคร
JOKER GAMING เป็นผู้ให้บริการเกมสล็อตที่มีชื่อเสียง และได้การยอมรับอย่างกว้างขวางในวงการ มากกว่า 10 ปี ได้สร้างชื่อให้กับตัวเองในฐานะผู้ให้บริการเกมสล็อตชั้นนำและเชื่อถือได้ สำหรับผู้เล่นในประเทศไทยและทั่วโลก รับประกันว่าผู้เล่นจะเพลิดเพลินไปกับเกมได้อย่างมั่นใจโดยไม่ต้องกังวล เล่นเกมสล็อต ทดลอง คุณภาพสูงที่ผู้ให้บริการรายอื่นไม่สามารถเทียบได้ ด้วยความมุ่งมั่นในความเป็นเลิศและการเน้นที่คุณภาพ จึงโดดเด่นเหนือใครในฐานะตัวเลือกอันดับต้น ๆ ของผู้ที่ชื่นชอบเกมสล็อต
ทำไมการเล่นเกมสล็อตบนสล็อตเว็บตรง จึงได้รับความนิยมเป็นอย่างมาก ขอแนะนำ 3 เหตุผลต่อไปนี้ ที่จะตอบได้ตรงคำถาม โดยเว็บสล็อตแห่งนี้สามารถตอบสนองความต้องการของผู้เล่นทุกคนได้ ด้วยบริการสล็อตฟรีที่มีคุณภาพสูงสุด นั่นเป็นเพราะ เว็บตรง สล็อต ฝากถอน ไม่มี ขั้นต่ำ 1 บาท ก็ ถอนได้ วอ เลท สามารถดึงดูดนักพนันจากทั่วทุกมุมโลก ให้มาใช้บริการอย่างมากมายทุกวัน มั่นใจได้ว่าหากคุณใช้เว็บนี้ คุณจะมีความสนุกสนานทุกวันแน่นอน
สล็อตเว็บตรง มีการอัปเดตเกมอย่างต่อเนื่อง โดยมีเกมสล็อตใหม่ ๆ มากมายให้ผู้เล่นเลือกเล่น รวมกว่า 500 เกม นอกจากนี้ เว็บตรง 100 ไม่มี ขั้นต่ำ ยังมีผู้ให้บริการเกมยอดนิยมที่มีเกมเว็บสล็อตใหม่ล่าสุดให้เลือกเล่นหลากหลาย ให้ได้เพลิดเพลินอย่างเต็มที่ ผู้ใช้สามารถเข้าถึงและเล่นเกมใดก็ได้ตามที่ต้องการ
เว็บแท้ จะพาคุณไปพบกับเกมสล็อตใหม่ล่าสุดที่มีอัตราการจ่ายเงินรางวัลสูง ไม่ว่าคุณจะเลือกผู้ให้บริการเกมรายใด คุณมีโอกาสที่จะชนะได้แบบกำไรไม่จำกัด มั่นใจได้ว่าเมื่อคุณเริ่มใช้บริการ คุณจะสามารถสร้างรายได้อย่างแน่นอน เล่นเกมสล็อตออนไลน์กับเว็บตรงและสัมผัสกับความตื่นเต้นของการคว้ารางวัลใหญ่ได้เลย!
เว็บสล็อต ให้ผู้เล่นทุกคนสามารถปรับการเดิมพันในเกมสล็อตได้ โดยไม่มีข้อจำกัดด้านจำนวนเงิน คุณสามารถวางเดิมพันได้ตั้งแต่ขั้นต่ำ 1 บาท ไปจนถึง 100 บาทในครั้งเดียว ไม่ว่าคุณจะเป็นนักพนันทั่วไปหรือผู้เล่นระดับไฮโรลเลอร์ คุณสามารถเพลิดเพลินไปกับการเล่นเกมสล็อตที่ชนะง่ายและจ่ายสูง บนสล็อต เว็บ ตรงที่มีคุณภาพได้ตลอดทั้งวัน เพื่อความสนุกและความตื่นเต้นสูงสุด
สัมผัสความบันเทิงขั้นสุดยอดที่ดึงดูดใจผู้ที่ชื่นชอบเกมสล็อต ให้เข้ามาเดิมพันบน สล็อตเว็บตรง อย่างต่อเนื่อง ส่วนหนึ่งมาจากการคัดสรรเกมสล็อตแท้มาอย่างพิถีพิถัน และนำเข้าโดยตรงจากต่างประเทศ รับรองว่าคุณจะได้รับความบันเทิงชั้นยอดบนเว็บ สล็อต ใหม่ ล่าสุด ชนะได้ง่าย ไม่มีขีดจำกัด ผู้ให้บริการเกมแต่ละรายทุ่มเทเพื่อผลิตเกมสล็อตคุณภาพสูงและครอบคลุมเต็มรูปแบบ เพื่อตอบสนองนักพนันรุ่นใหม่ ทำให้สนุกสนานยิ่งขึ้น ผู้ให้บริการแต่ละรายได้รับอนุญาตให้เล่นสล็อตตามกฎหมาย และสร้างเกมสล็อตที่มีสัญลักษณ์พิเศษหลากหลาย มาเล่นแล้วรับโบนัสเพิ่มเติมได้เลย!
สำหรับผู้เล่นเกมสล็อต เว็บ ตรง 100 อย่าพลาดกับผู้ให้บริการยอดนิยมที่มีชื่อเสียง ให้ความสนุกสนานและน่าตื่นตาตื่นใจ ผู้ให้บริการเกมสล็อตชั้นนำหลายรายให้บริการมายาวนาน ด้วยคุณสมบัติที่โดดเด่นและแตกโบนัสได้ต่อเนื่อง ทำให้เป็นตัวเลือกอันดับต้น ๆ ของผู้ที่ชื่นชอบการเล่นเกมสล็อต เว็บตรง เป็นเกมที่เล่นง่าย ให้เงินรางวัลรวดเร็ว และมีระดับความยาก-ง่ายแตกต่างกันออกไป รองรับผู้เล่นทั้งมือใหม่และมืออาชีพ รวมเกมฮิตไว้บนเว็บตรงชั้นนำ พร้อมรักษาความสนุกในสไตล์คลาสสิกให้ผู้เล่นเพลิดเพลินได้อย่างต่อเนื่อง ให้กราฟิกที่สวยงามและน่าจดจำ ซึ่งไม่ซ้ำใครและมาพร้อมโบนัสสุดคุ้ม!
สล็อตเว็บตรง บริการช่องทางเข้าเล่นเกมสล็อตจากเว็บแท้ ซึ่งสามารถเข้าถึงได้ง่าย ผ่านอุปกรณ์พกพาของทุกระบบปฏิบัติการ มั่นใจได้ว่าคุณจะเพลิดเพลินไปกับการหมุนสล็อตด้วยระบบอัตโนมัติที่ดีที่สุด ช่วยให้คุณสนุกไปกับการเล่นเกมบนเว็บได้ตลอดทั้งวัน ไม่มีสะดุดนอกจากนี้ เว็บยังมีระบบปฏิบัติการที่มีความเสถียรสูง ทำให้ทุกคนสามารถเล่นเกมสล็อต บนเว็บตรงได้อย่างราบรื่น ไม่มีการหยุดชะงัก ดังนั้น สำหรับนักพนันที่กำลังมองหาแพลตฟอร์มชั้นนำ สำหรับการหมุนสล็อต pg เว็บตรง แตกหนัก และตอบสนองความต้องการ แนะนำมาสมัครกับเว็บตรงเท่านั้น คุณจะประทับใจ ไม่ว่าคุณจะใช้โทรศัพท์มือถือระบบ Android หรือ iOS คุณก็สามารถลงทุนและเข้าร่วมเล่นเกมทำเงินได้อย่างมั่นใจ
ตอบ : เพียงลงทะเบียนสมัครเว็บสล็อต คุณจะสามารถเข้าถึงเกมสล็อตหลากหลายประเภท มีให้เลือกเล่นมากกว่า 1,000 เกม แต่ละเกมสล็อต มาพร้อมกับกราฟิกที่สวยงาม ภาพคมชัดระดับ Full HD รับรองว่าคุณจะได้สัมผัสกับการเล่นเกมที่สนุกและสมจริง มั่นใจได้ว่าคุณจะได้รับความพึงพอใจสูงสุด
ตอบ: การเข้าสู่ระบบในเว็บสล็อตเป็นขั้นตอนง่าย ๆ ไม่ยุ่งยาก ใช้เวลาน้อยกว่า 3 วินาที ไม่เพียงแต่จะง่ายเท่านั้น แต่ยังมีจุดเข้าใช้งานได้หลายจุด คุณสามารถเลือกวิธีที่สะดวกที่สุดในการเข้าถึงสล็อตแท้ได้เลย
ตอบ: มั่นใจได้ว่าให้บริการฝากและถอนเงินบนสล็อต เว็บตรง ไม่มีขั้นต่ำ ด้วยเงินเพียง 1 บาท, 10 บาท หรือ 100 บาท คุณสามารถฝากและถอนได้อย่างง่ายดาย วอเลท บนเว็บสล็อต และไม่มีการเรียกเก็บค่าธรรมเนียมแม้แต่บาทเดียว